Join the conversation

Done
Reply
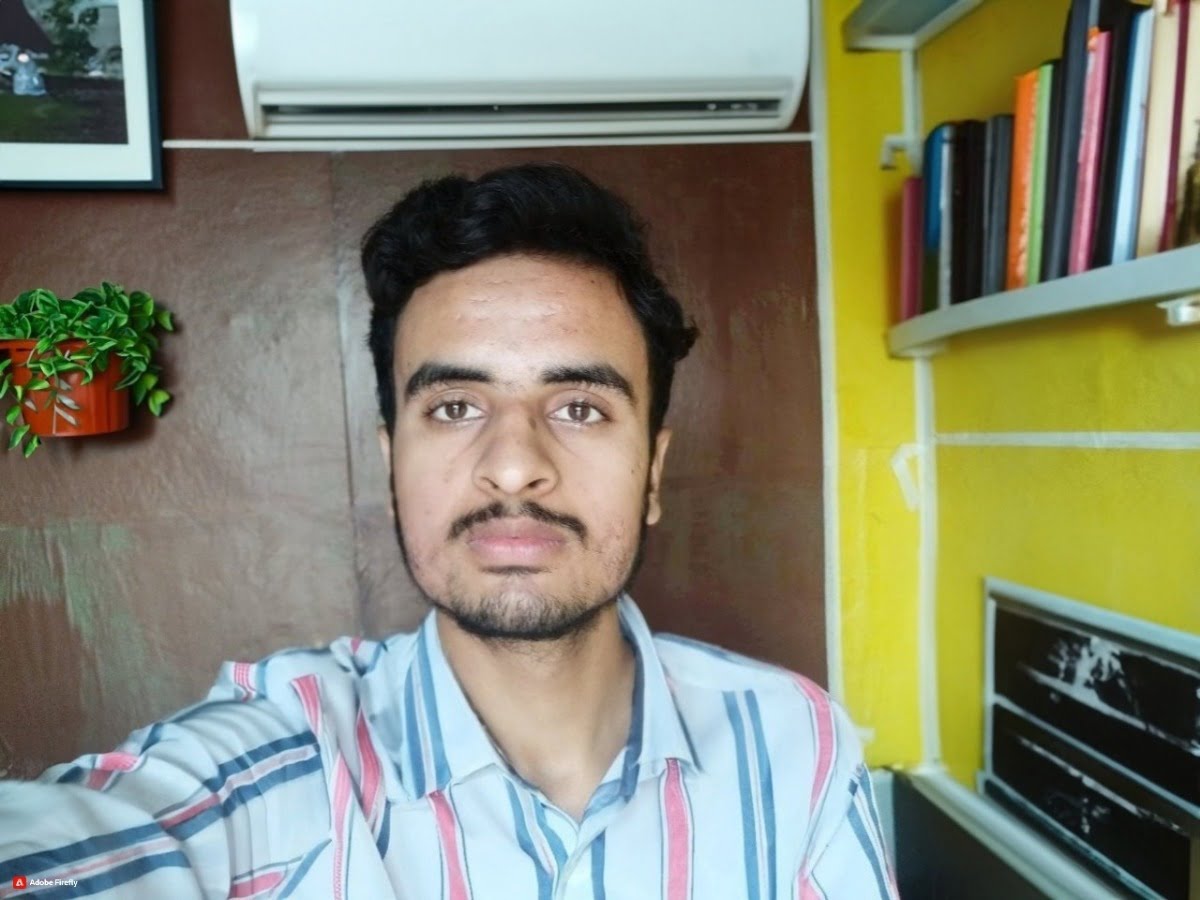
Done
Reply
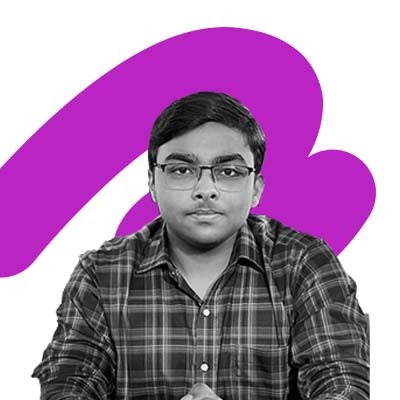
This is code with Titanic data set:import pandas as pd
import numpy as np
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import seaborn as sns# Load the Titanic dataset (assuming it's in a CSV file named 'titanic.csv')
titanic_data = sns.load_dataset("titanic")# Preprocess the data
# Let's consider 'Age' and 'Fare' as predictor variables and 'Survived' as the target variable
X = titanic_data[['age', 'fare']]
y = titanic_data['survived']# Handle missing values
X.fillna(X.mean(), inplace=True)# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)# Perform polynomial feature transformation
poly_features = PolynomialFeatures(degree=2)
X_train_poly = poly_features.fit_transform(X_train)
X_test_poly = poly_features.transform(X_test)# Fit the polynomial regression model
poly_reg = LinearRegression()
poly_reg.fit(X_train_poly, y_train)# Make predictions
y_train_pred = poly_reg.predict(X_train_poly)
y_test_pred = poly_reg.predict(X_test_poly)# Evaluate the model
train_rmse = np.sqrt(mean_squared_error(y_train, y_train_pred))
test_rmse = np.sqrt(mean_squared_error(y_test, y_test_pred))print("Train RMSE:", train_rmse)
print("Test RMSE:", test_rmse)
Reply

I have done this lecture with 100% practice.
Reply

python code for polynomial regression line drawing
Reply

AIC and BIC are statistical measures used in model selection and comparison in the field of statistics and machine learning. Both AIC (Akaike Information Criterion) and BIC (Bayesian Information Criterion) are used to evaluate the goodness of fit of different models based on the data and penalize overly complex models.